JavaScript: Useful number formatting functions
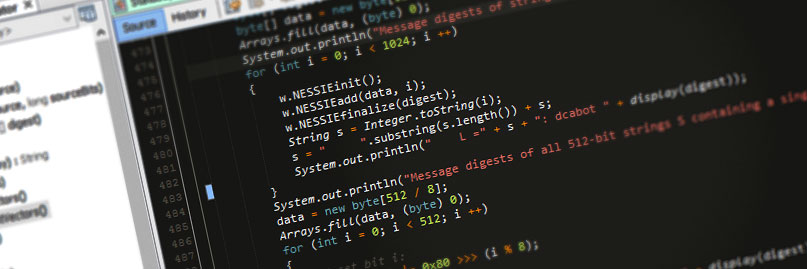
Recently I’ve been developing a lot of reporting applications in JavaScript and have collected a few helper functions that I use regularly for number formatting. Here’s a look at each function and how you can use it in your own projects.
Rounding a number to a defined decimal precision
Input: 1234.567
Output: 1234.6 (to one decimal precision)
Usage:
var myNumOld = 1234.567 var myNumNew = numberPrecision(myNumOld, 1)
Function:
/** * Returns a rounded number to the precision specified. * @author Darian Cabot * @see https://www.dariancabot.com * @param {Number} value A number to be rounded. * @param {Number} decimals The number of decimal places to round to. * @returns the value rounded to the number of decimal places specified. * @type Number */ function numberPrecision(value, decimals) { return Math.round(value * Math.pow(10, decimals)) / Math.pow(10, decimals); }
Adding commas to a number to break up thousands
Input: 1234.567
Output: 1,234.567
Usage:
var myNumOld = 1234.567 var myNumNew = addCommas(myNumOld)
Function:
/** * Formats a numeric string by adding commas for cosmetic purposes. * @author Keith Jenci * @see http://www.mredkj.com/javascript/nfbasic.html * @param {String} nStr A number with or without decimals. * @returns a nicely formatted number. * @type String */ function addCommas(nStr) { nStr += ''; var x = nStr.split('.'); var x1 = x[0]; var x2 = x.length > 1 ? '.' + x[1] : ''; var rgx = /(\d+)(\d{3})/; while (rgx.test(x1)) { x1 = x1.replace(rgx, '$1' + ',' + '$2'); } return x1 + x2; }
Adding an ordinal for counting numbers
Input: 23
Output: 23rd
Usage:
var myNumOld = 23 var myNumNew = myNumOld.toOrdinal()
Function:
/** * Adds an ordinal to a number (e.g. 'st', 'nd', 'rd', 'th'). * @author Venkat K * @see http://www.eggheadcafe.com/community/aspnet/3/43489/hi.aspx * @param {Number} A positive number. * @returns a the original number and a ordinal suffix. * @type String */ Number.prototype.toOrdinal = function() { var n = this % 100; var suffix = ['th', 'st', 'nd', 'rd', 'th']; var ord = n < 21 ? (n < 4 ? suffix[n] : suffix[0]) : (n % 10 > 4 ? suffix[0] : suffix[n % 10]); return this + ord; }